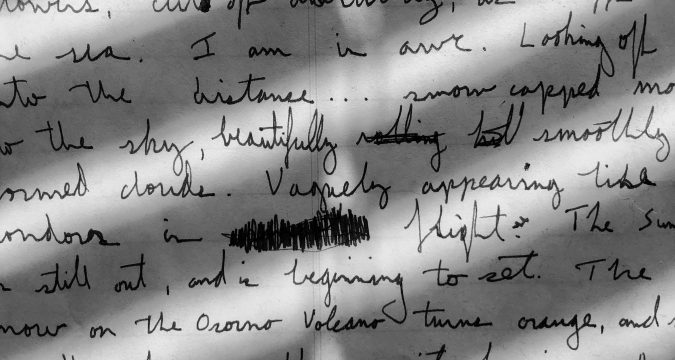
I’m working on a project that requires storing large multiline chunks of text in MongoDB. The chunks should be inserted in the database during initial database population, so I need to enter them somewhere in db population script. Unfortunately you can’t just put something like this:
db.tests.insert( {
name: 'test',
text: 'this is
my superawesome
multiline content'
} )
Javascript/JSON do not support multiline text like this. Fortunately there are several options that made my life easier. Here they are:
- Use Concatenation. Something like
db.tests.insert( {
name: 'test1',
text: 'this is ' +
'my superawesome ' +
'multiline content'
} )
Note that in this method even though you can enter long text it will be still single line. In order to store the text as multiline use the next method. - Encode control characters
db.tests.insert( {
name: 'test2',
text: "this is \nmy superawesome \nmultiline content"
} )
This method really stores multiple lines and you can even combine it with the previous method to make it look like multiline in your editor. Still, highly annoying, here is a better way: - Read from file
db.tests.insert( {
name: 'test2',
text: cat( 'my_file_with_large_multiline_content' )
} )
Now open file called my_file_with_large_multiline_content and put this into the file:
this is
my superawesome
multiline content
Now you can edit the file any way you want and MongoDB’s cat() will read the file contents and put its contents into your document. Yay!